SourceBuilder
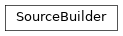
SourceBuilder inheritance diagram
- class savant.client.SourceBuilder(socket=None, log_provider=None, retries=3, module_health_check_url=None, module_health_check_timeout=60, module_health_check_interval=5, telemetry_enabled=False)
Builder for Source.
Usage example:
source = ( SourceBuilder() .with_log_provider(JaegerLogProvider('http://localhost:16686')) .with_socket('req+connect:ipc:///tmp/zmq-sockets/input-video.ipc') # Note: healthcheck port should be configured in the module. .with_module_health_check_url('http://172.17.0.1:8888/healthcheck') .build() ) result = source(JpegSource('cam-1', 'data/AVG-TownCentre.jpeg')) result.logs().pretty_print()
Usage example (async):
source = ( SourceBuilder() .with_log_provider(JaegerLogProvider('http://localhost:16686')) .with_socket('req+connect:ipc:///tmp/zmq-sockets/input-video.ipc') .build_async() ) result = await source(JpegSource('cam-1', 'data/AVG-TownCentre.jpeg')) result.logs().pretty_print()
- with_socket(socket)
Set ZeroMQ socket for Source.
- with_log_provider(log_provider)
Set log provider for Source.
- with_retries(retries)
Set number of retries for Source.
Source retries to send message to ZeroMQ socket if it fails.
- with_module_health_check_url(url)
Set module health check url for Source.
Source will check the module health before receiving any messages.
- with_module_health_check_timeout(timeout)
Set module health check timeout for Source.
Source will wait for the module to be ready for the specified timeout.
- with_module_health_check_interval(interval)
Set module health check interval for Source.
Source will check the module health every specified interval.
- with_telemetry_enabled()
Enable telemetry for Source.
- with_telemetry_disabled()
Disable telemetry for Source.
- build()
Build Source.
- build_async()
Build async Source.