SinkBuilder
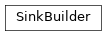
SinkBuilder inheritance diagram
- class savant.client.SinkBuilder(socket=None, log_provider=None, idle_timeout=None, module_health_check_url=None, module_health_check_timeout=60, module_health_check_interval=5, source_id=None, source_id_prefix=None)
Builder for Sink.
Usage example:
sink = ( SinkBuilder() .with_socket('rep+connect:ipc:///tmp/zmq-sockets/output-video.ipc') .with_idle_timeout(60) .with_log_provider(JaegerLogProvider('http://localhost:16686')) # Note: healthcheck port should be configured in the module. .with_module_health_check_url('http://172.17.0.1:8888/healthcheck') .build() ) for result in sink: print(result.frame_meta) result.logs().pretty_print()
Usage example (async):
sink = ( SinkBuilder() .with_socket('rep+connect:ipc:///tmp/zmq-sockets/output-video.ipc') .with_idle_timeout(60) .with_log_provider(JaegerLogProvider('http://localhost:16686')) .build_async() ) async for result in sink: print(result.frame_meta) result.logs().pretty_print()
- with_socket(socket)
Set ZeroMQ socket for Sink.
- with_log_provider(log_provider)
Set log provider for Sink.
- with_idle_timeout(idle_timeout)
Set idle timeout for Sink.
Sink will stop trying to receive a message from ZeroMQ socket when it did not receive a message for idle_timeout seconds.
- with_module_health_check_url(url)
Set module health check url for Sink.
Sink will check the module health before receiving any messages.
- with_module_health_check_timeout(timeout)
Set module health check timeout for Sink.
Sink will wait for the module to be ready for the specified timeout.
- with_module_health_check_interval(interval)
Set module health check interval for Sink.
Sink will check the module health every specified interval.
- with_source_id(source_id)
Set source id for Sink.
Sink will filter messages by source id.
- with_source_id_prefix(source_id_prefix)
Set source id prefix for Sink.
Sink will filter messages by source id prefix.
Note: source_id and source_id_prefix are mutually exclusive. If both are set, source_id will be used.
- build()
Build Sink.
- build_async()
Build async Sink.